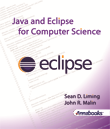
Print Price: $39.95
|
By Sean D. Liming and John R. Malin
Practical Textbook for Learning Java Programming,
Computer Science, and Eclipse
Almost every job today has some
interaction with a computer or a computing device.
Computers come in all shapes and sizes such as
smartphones, ATM machines, thermostats, test equipment,
robotics, point-of-sale systems, cloud servers, projection
systems, and, oh yes, personal computers. All of them need
to be designed, built, and programmed. Having a good
understanding of computer programming and Computer Science
can provide a good foundation for one’s career. The Java
programming language is one of the most popular
programming languages used today. By learning Java, you
will have a good understanding of structured programming,
and Java is a good vehicle to learn the basics of Computer
Science.
Employers are always looking for
new-hires to have practical experience. The best way to
stand out during the interview process is to demonstrate
that you have a familiarity with the tools used by
professional programmers. There are many Java development
tools available, but when it comes to Java programming,
Eclipse is the tool frequently used in the industry.
Eclipse is a popular Integrated Development Environment
(IDE) that supports Java, C/C++, and web development.
The goal of this textbook is to combine
Java programming, Computer Science, and a popular
development tool that not only prepares you for the
Computer Science curriculum but also beyond the classroom
into your professional career. The 14 chapters start with
the basics of how Algebra flows into computer programming,
moves on to logical program flow, and then to Object
Oriented Programming. After these fundamentals come the
advanced topics of recursion, search, sort, and Big-O
notation. Going beyond the basic curriculum material, the
later chapters cover graphical programming with JavaFX,
File I/O, an introduction to data structures, and finishes
with JavaFX 2-D Game development. There are many computer
activities to provide a hands-on experience and keep you
involved during the reading of this book.
|
|
1 The Future Starts Now
1.1 Our Connected World
1.2 Computer Activity 1.1: Computing Ideas Spread
Throughout Human History
1.3 The 1s and 0s that make Computers Work
1.4 Computer Architecture
1.5 The Evolution of Computer Programming
1.6 A Very Brief History of Java
1.7 Compiler versus Interpreter
1.8 Computer Science
1.9 AP® Computer Science Exam and About this Book
1.10 Summary and Homework Assignments
2 Eclipse and Objects
2.1 Why Eclipse
2.2 Computer Activity 2.1: JDK and Eclipse Download and
Installation
2.3 Computer Activity 2.2: First Application
2.4 Structured Programming and Object-Oriented Programming
(OOP) Brief Introduction
2.5 Java Virtual Machine (JVM), a High-Level Description
2.6 Eclipse Feature: Searching
2.7 Eclipse Feature: Moving Project from One System to
Another
2.8 Summary and Homework Assignments
3 Math and Strings
3.1 Data Types and Math Operators
3.2 Math Methods
3.3 Variables and Constants
3.4 Random Number Generator
3.5 Strings
3.6 Getting User Input
3.7 Comments in Code
3.8 Summary and Homework Assignments
4 Controlling the Program Flow and Iteration
4.1 Flowchart Diagram
4.2 If-Else Statement, Relational Operators, and Boolean
Expressions
4.3 Nested If-Else
4.4 If-Else-If Ladder
4.5 Switch-Case
4.6 Loops / Iteration
4.7 Eclipse Feature: Flowchart Add-On
4.8 Scope of Variables
4.9 Eclipse Feature: Debugging
4.10 Summary and Homework Assignments
5 Arrays
5.1 Arrays and the One-dimensional Arrays
5.2 Two-dimensional Arrays
5.3 The ArrayList
5.4 Summary and Homework Assignments
6 Methods, Classes, and Packages
6.1 Creating Methods
6.2 Creating and Using Classes: Encapsulation and
Instantiation
6.3 Eclipse Reference Icons
6.4 Packages and Jar Files
6.5 Javadoc and Eclipse
6.6 Summary and Homework Assignments
7 Inheritance and Polymorphism
7.1 Inheritance
7.2 The Object Class
7.3 Inheritance with Constructors and Class Access
7.4 Eclipse Feature: Quickly Creating Constructors
7.5 Polymorphism
7.6 Eclipse Feature: Quickly Adding Overrides
7.7 Architecting A Library: Abstract Class and Interface
Type
7.8 Java Library Subset for AP® Computer Science and the
List Interfaces
7.9 Documenting the Library with Unified Modeling Language
(UML) – Class Diagrams
7.10 Eclipse Feature: UML Add-Ons
7.11 Summary and Homework Assignments
|
8 Software Development, Exception Handling, and Other
Debug Techniques
8.1 Software Design Process and Regulations
8.2 Code Standards – “Defensive Coding”
8.3 Eclipse Feature: Code Refactoring
8.4 Exception Handling
8.5 Assertions
8.6 Eclipse Feature: VisualVM Add-on
8.7 The Very Deep End: Java-to-Assembly
8.8 Summary and Homework Assignments
9 Recursion
9.1 Computer Activity 9.1 - Factorial Recursion Style
9.2 Computer Activity 9.2 - Debugging Recursion in Eclipse
9.3 Computer Activity 9.3 - Iteration versus Recursion
9.4 Computer Activity 9.4 - Mutual Recursion
9.5 Tail Recursion
9.6 Computer Activity 9.5 - Private Recursive Helper
Method
9.7 A Real-World Example
9.8 Summary and Homework Assignments
10 Sort and Search with Introduction to Algorithm
Analysis
10.1 Algorithm Analysis Introduction – Big-O Notation
10.2 Computer Activity 10.1 - Sorting Algorithms
10.3 Computer Activity 10.2 - Searching Algorithms
10.4 Algorithm Analysis – The Final Picture
10.5 Summary and Homework Assignments
11 Introduction to GUI Programming with Swing and JavaFX
11.1 AWT and Swing
11.2 JavaFX
11.3 Summary and Homework Assignments
12 File I/O using the Stream Classes
12.1 Java’s Stream I/O
12.2 Reading and Writing with Byte Streams
12.3 Auto File Close with Try-with-Resource
12.4 Saving Other Primitive Data Types using
DataInputStream and DataOutputStream
12.5 Saving Objects
12.6 Random Access File
12.7 Reading and Writing with Character Streams
12.8 Summary and Homework Assignments
13 Introduction to Data Structures: the Collections
Framework
13.1 The Collections Framework
13.2 List Interface
13.3 Set Interface
13.4 Queue Interface
13.5 Map Interface
13.6 Collections Class Algorithms
13.7 Putting it all Together: Data Structures and File I/O
13.8 Computer Activity 13.7 – JavaFX ListView and ComboBox
Controls
13.9 Summary and Homework Assignments
14 JavaFX 2D Games
14.1 JavaFX Multimedia and Game Engines
14.2 Media Content Production Software
14.3 JavaFX Drawing, Import Graphics, and Text
14.4 Animation and the Game Loop
14.5 Keyboard and Mouse Controls / Input Events
14.6 Sound and Music Playback
14.7 Computer Activity 14.6 - Street Frog
14.8 Computer Activity 14.7 - Tie Attack!
14.9 Eclipse Feature: Export Runnable JAR File
14.10 Eclipse Feature: Clone a Project for a New Version
14.11 Summary
|